Making Store Purchases with the SKStoreProductViewController Class
Previous | Table of Contents | Next |
An iOS 6 iPhone Twitter Integration Tutorial using SLRequest | Building In-App Purchasing into iPhone iOS 6 Applications |
Learn SwiftUI and take your iOS Development to the Next Level |
For quite some time, the iOS SDK has included the Store Kit Framework, the purpose of which is to enable applications to implement what are referred to as “in-app purchases”. This typically provides a mechanism for application developers to charge users for additional items and services over and above the initial purchase price of the application. Typical examples include purchasing access to higher levels in a game or a monthly subscription to premium content.
The Store Kit Framework has also traditionally provided the ability to enable users to purchase items from Apple’s iTunes, App and iBook stores. Prior to the introduction of iOS6, the Store Kit Framework took the user out of the current application and into the iTunes application to initiate and complete an iTunes Store purchase. iOS 6, however, introduces a new view controller class (SKStoreProductViewController) which presents the store product directly within the application using a pre-configured view. This chapter will provide an overview of the SKStoreProductViewController class before working through an example implementation of this class in an iPhone application.
The SKStoreProductViewController Class
The SKStoreProductViewController class makes it possible to integrate purchasing from Apple’s iTunes, App and iBooks stores directly into iOS 6 applications with minimal coding work. The developer of a music review application, might, for example want to provide the user with the option to purchase an album from iTunes after reading a review in the application. The developer of multiple applications may want to encourage users of one of those applications to purchase related applications.
All that is required to implement the SKStoreProductViewController functionality is to initialize an instance of the class with an item ID for the product to be purchased from the store and then to load the product details. Once product details have been loaded, the view controller is presented to the user.
Product item IDs can be obtained a number of ways. For specific items, the ID can be obtained by locating the product in iTunes and Ctrl-clicking (or right-clicking on Windows) on the product image. In the resulting menu, select the option to copy the URL. Paste the URL into a text editor and extract the ID from the end. The ID from the following URL, for example, is 527049179.
http://itunes.apple.com/us/movie/the-pirates!-band-of-misfits/id527049179
Alternatively, searches may be performed on a variety of criteria using the Apple Search API, details of which can be found at the following URL:
When the user has finished in the store view, a delegate method is called to notify the application, at which point the store view controller can be dismissed. The remainder of this chapter will work through the creation of a simple example application to demonstrate the use and implementation of the SKStoreProductViewController class.
Creating the Example Project
The example application created in this chapter will consist of a single button which, when touched, will display the store kit view controller primed with a specified product.
Launch Xcode and create a new project by selecting the options to create a new iPhone iOS application based on the Single View Application template. Enter StoreKitDemo as the product name and class prefix, set the device to iPhone and select the Use Storyboards and Use Automatic Reference Counting options if they are not already selected.
Creating the User Interface
Within the project navigator panel, select the MainStoryboard.storyboard file and drag and drop a Button from the Object Library to the center of the view. Double click on the button text and change it to “Buy Now” so that the layout matches Figure 74-1.
Figure 74-1
Display the Assistant Editor and make sure it is showing the code for the StoreKitDemoViewController.h file. Select the button in the view and then Ctrl-click on it and drag the resulting line to a location just beneath the @interface line in the Assistant Editor panel. Release the line and in the resulting panel change the connection type to Action and name the method showStoreView. Click on the Connect button and close the Assistant Editor panel.
Displaying the Store Kit Product View Controller
When the user touches the Buy Now button, the SKStoreProductViewController instance needs to be created, configured and displayed. Before writing this code, however, it will be necessary to declare the StoreKitDemoViewController class as implementing the <SKStoreProductViewControllerDelegate> protocol. The <StoreKit/StoreKit.h> file also needs to be imported. Both of these tasks need to be performed in the StoreKitDemoViewController.h file which should be modified to read as follows:
#import <UIKit/UIKit.h> #import <StoreKit/StoreKit.h> @interface StoreKitDemoViewController : UIViewController <SKStoreProductViewControllerDelegate> - (IBAction)showStoreView:(id)sender; @end
Within the StoreKitDemoViewController.m file, locate the stub showStoreView method and implement the code as outlined in the following listing:
- (IBAction)showStoreView:(id)sender { SKStoreProductViewController *storeViewController = [[SKStoreProductViewController alloc] init]; storeViewController.delegate = self; NSDictionary *parameters = @{SKStoreProductParameterITunesItemIdentifier: [NSNumber numberWithInteger:333700869]}; [storeViewController loadProductWithParameters:parameters completionBlock:^(BOOL result, NSError *error) { if (result) [self presentViewController:storeViewController animated:YES completion:nil]; }]; }
The code begins by creating and initializing a new SKStoreProductViewController instance:
SKStoreProductViewController *storeViewController = [[SKStoreProductViewController alloc] init];
Next, the view controller class assigns itself as the delegate for the storeViewController instance:
storeViewController.delegate = self;
An NSDictionary instance is then created and initialized with a key of SKStoreProductParameterITunesItemIdentifier associated with an NSNumber value representing a product in a Store (in this case an album in the iTunes store).
NSDictionary *parameters = @{SKStoreProductParameterITunesItemIdentifier: [NSNumber numberWithInteger:333700869]};
Finally, the product is loaded into the view controller and, in event that the load was successful, the view controller is presented to the user:
[storeViewController loadProductWithParameters:parameters completionBlock:^(BOOL result, NSError *error) { if (result) [self presentViewController:storeViewController animated:YES completion:nil]; }];
Implementing the Delegate Method
All that remains is to implement the delegate method that will be called when the user has either completed or cancelled the product purchase. Since the StoreKitDemoViewController class was designated as the delegate for the SKStoreProductViewController instance, the method needs to be implemented in the StoreKitDemoViewController.m file:
#pragma mark - #pragma mark SKStoreProductViewControllerDelegate -(void)productViewControllerDidFinish:(SKStoreProductViewController *)viewController { [viewController dismissViewControllerAnimated:YES completion:nil]; }
For the purposes of this example, all the method needs to do is to tell the view controller to dismiss itself.
Adding the Store Kit Framework to the Build Phases
Within the project navigator panel, select the StoreKitDemo target at the top of the list and in the main panel select the Build Phases tab. In the Link Binary with Libraries category, click on the + button and in the resulting list of libraries search for and add the StoreKit.framework library.
Testing the Application
Build and run the application either on a physical iPhone device or using the iOS Simulator. Touch the button and wait until the store kit product view controller appears as illustrated in Figure 74-2. Note that there may be a short delay while the store kit contacts the iTunes store to request and download the product information.
Touching the Cancel button should trigger the delegate, dismissing the view controller and returning the user to the original view controller screen containing the button.
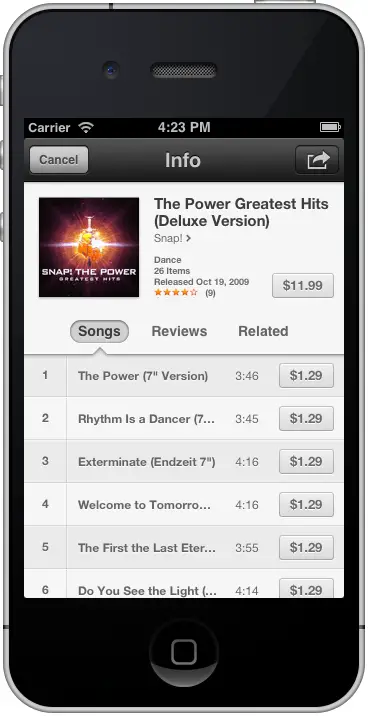
Figure 74-2
Edit the code for the showStoreView: method and modify the item ID from 333700869 (an iTunes Store product) with 435744790 (an iBook Store product). Re-run the application and note that the view controller class has changed layout automatically to accommodate a book product (Figure 74 3).
Figure 74-3
The class, will, of course, adapt similarly when passed the ID of an app or movie.
Summary
The SKStoreProductViewController class is new to iOS 6 and provides an easy and visually appealing interface for allowing Apple-based store purchases such as movies, books, music and apps to be made directly from within an iOS application. As demonstrated, this functionality can be added to an application with a minimal amount of code.
Learn SwiftUI and take your iOS Development to the Next Level |
Previous | Table of Contents | Next |
An iOS 6 iPhone Twitter Integration Tutorial using SLRequest | Building In-App Purchasing into iPhone iOS 6 Applications |